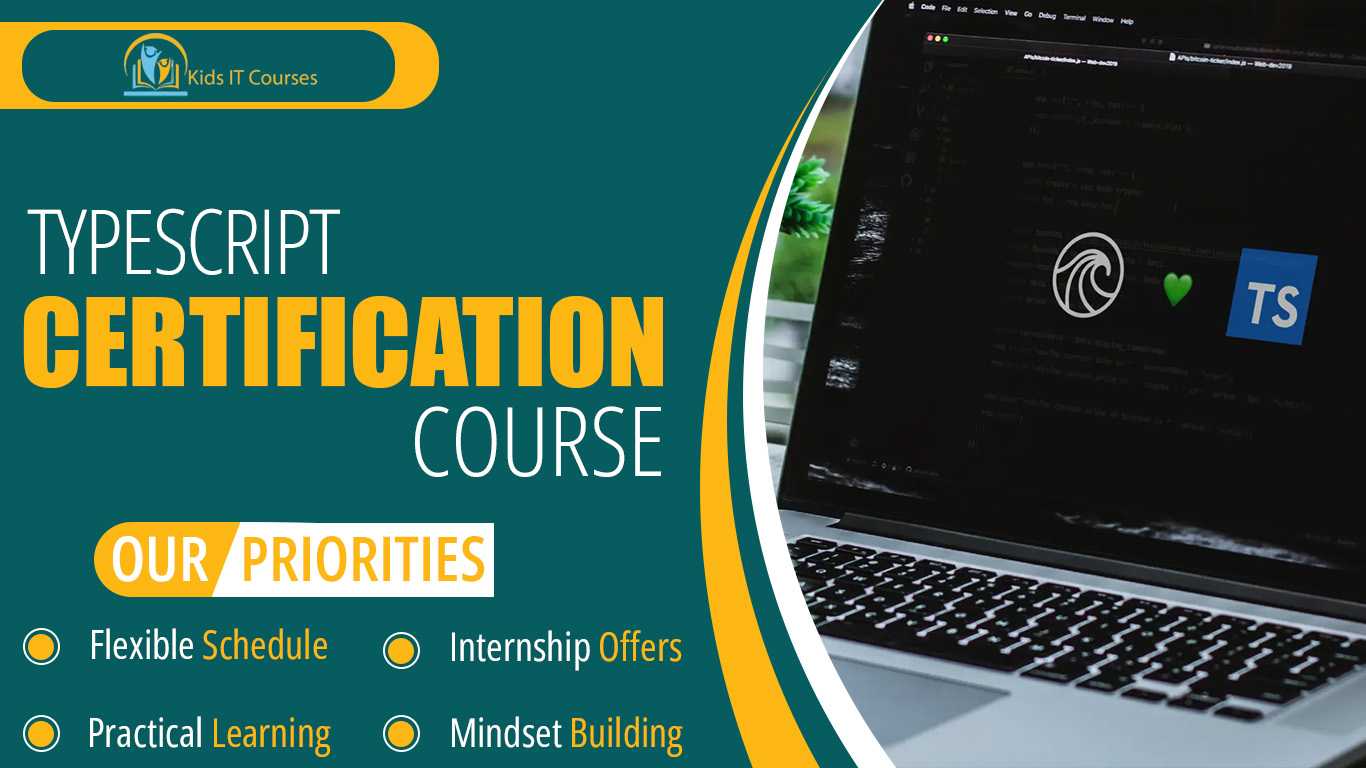
TypeScript Course for Kids
Definition
• TypeScript is a smart version of JavaScript. It helps kids write better and safer code.
• It tells kids if something is wrong while typing code. This helps them fix mistakes fast.
• If kids know JavaScript, TypeScript is easy. It just adds extra help and rules.
• TypeScript helps kids manage big apps or games. It keeps their code neat and organized.
• Kids learn how to plan better before they write code. It boosts their problem-solving skills too.
• Tech companies love TypeScript. Kids learn what professionals use!
Importance
• TypeScript helps kids write better and safer code. It shows mistakes before running the code.
• It is just like JavaScript but with extra help. If kids know JavaScript, learning TypeScript is super easy.
• Many big websites and apps use TypeScript. Kids get real tech skills for the future.
• It keeps code neat and easy to understand. Kids can manage big projects step by step.
• TypeScript shows where the problems are. Kids can fix errors quickly without guessing.
• Kids learn how to plan and think logically. It builds strong problem-solving habits.
• TypeScript is perfect for making web apps. It opens doors to other cool coding tools.
Advantages for Freelancing
• TypeScript helps kids find and fix errors quickly. It shows problems before running the code.
• TypeScript tells kids when they make a mistake. This helps them write better and safer code.
• TypeScript is like a smarter version of JavaScript. If kids know JS, they can learn TS easily.
• Kids can build apps, games, and websites with it. It’s used in big tech companies too!
• TypeScript finds problems before running the code. Kids save time and learn how to fix things faster.
• Kids can use it to make websites or games. It works well with tools they already use.
• Learning TypeScript gives kids a strong start. They feel ready to learn more advanced languages.
Session 1 : What is TypeScript?
Introduction to TypeScript as a superset of JavaScript
Real-life example: Making a large app safer and more maintainable
Key benefits: type safety, better tooling, fewer bugs
Installing TypeScript and setting up a basic project
Activity: Create and compile your first TypeScript file
Session 2 : Type Annotations & Variables
Understanding type annotations for variables
Declaring basic types: string, number, boolean, any
Real-life example: Validating user input with correct types
Activity: Build a form with typed fields and validation
Session 3 : Functions and Custom Types
Writing typed functions with return types and parameters
Creating custom types using
type
andinterface
Real-life example: A function that calculates invoice totals
Activity: Define and use custom types in a sample project
Session 4 : Classes and Object-Oriented Programming
Defining classes, constructors, and properties
Using inheritance and access modifiers (
public
,private
)Real-life example: Creating a
User
orProduct
classActivity: Build and extend a class for an online store
Session 5 : Arrays, Tuples & Enums
Typed arrays and using generic types like
Array<string>
Introduction to tuples and enum types for fixed options
Real-life example: Managing product categories or user roles
Activity: Create a shopping cart using arrays, enums, and tuples
Session 6 : Advanced Types and Utility Types
Working with union, intersection, and literal types
Using utility types:
Partial
,Readonly
,Pick
,Record
Real-life example: Flexible APIs with optional fields
Activity: Create complex data models for an app
Session 7 : Modules and Namespaces
Organizing code using ES modules and namespaces
Importing and exporting TypeScript components
Real-life example: Separating user logic and product logic in files
Activity: Break your code into modules and connect them
Session 8 : TypeScript with Frameworks
Using TypeScript with React, Node.js, or Angular
Setting up a TypeScript + Webpack project
Real-life example: TypeScript in a frontend or backend project
Activity: Build a small app using TypeScript + a framework
Bonus Materials
TypeScript cheat sheet and quick reference
Mini projects: Contact manager, Calculator, Blog post editor
Practice exercises with type challenges
Certificate of Completion for TypeScript Mastery